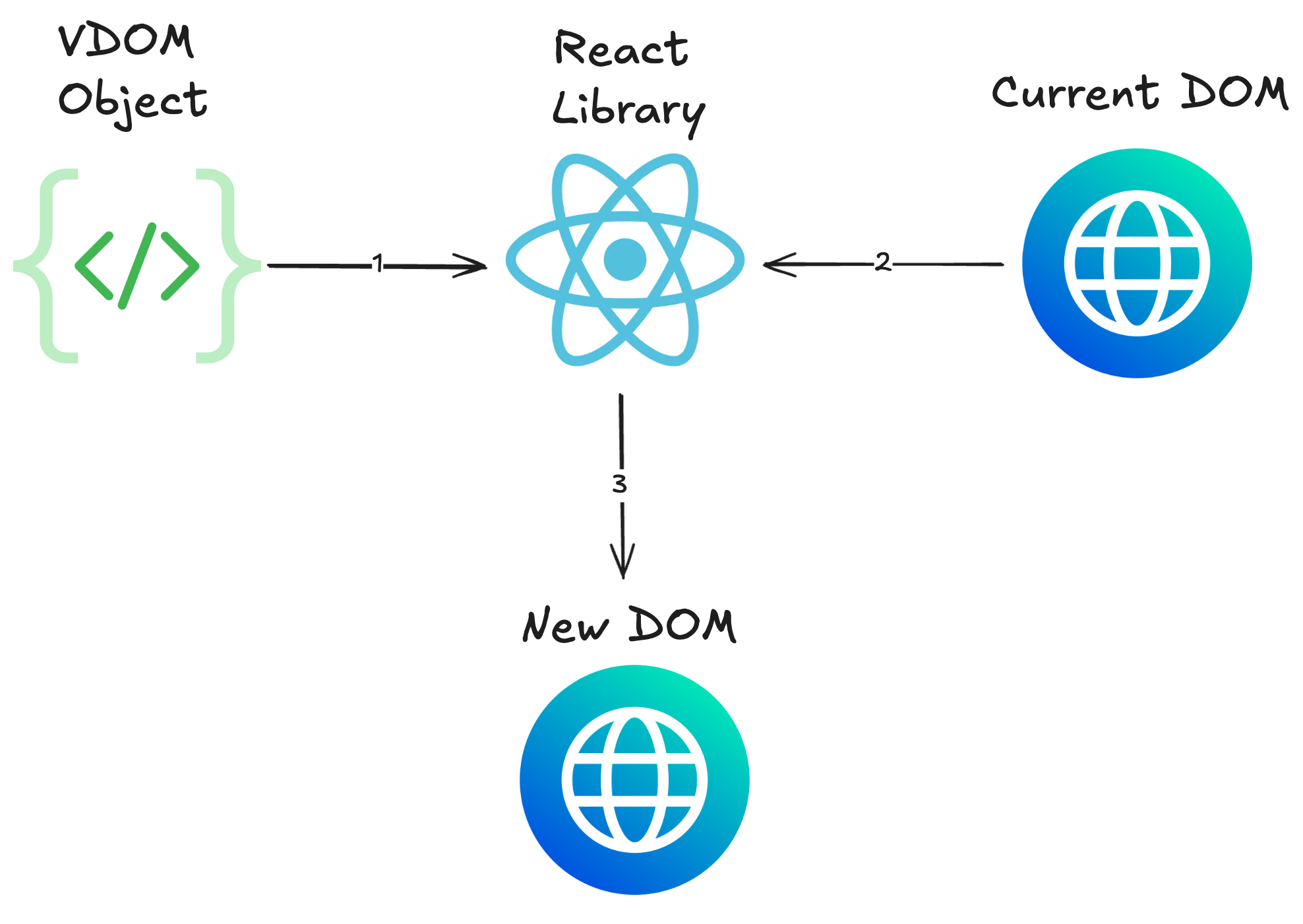
Understand the Virtual DOM in under 5 minutes
I began my career in software development as a self-taught web developer. My only motivation for learning React to begin with was to understand React Native, so I could then use it to make mobile apps. Now, I have been working as a professional web developer using React every day at work for a couple of years.
However, like many others, I never really took the time to understand how React actually works and why we even use it. So I decided to take a deep dive into the Virtual DOM, and I now believe that this understanding needs to be part of many web development curriculums.
The Virtual DOM is the centerpiece of React. If we wish to understand React, we must first understand the Virtual DOM.
In this article, I will explain the general workings of the Virtual DOM in simple terms so you can understand why it is important and why we use it.
What problem did React even fix?
Before React, web developers manually updated the DOM whenever the state of the application changed. This was an inefficient process, as the browser re-rendered the entire page every time a change occurred.
<div id="root"></div>
<script>
const root = document.getElementById("root");
const newDiv = document.createElement("div");
newDiv.id = "myDiv";
newDiv.style = "background-color: red";
newDiv.textContent = "This div was added using the DOM API";
root.appendChild(newDiv);
</script>
In the example above, we create a new <div>
element and append it to the #root
div using the DOM API and vanilla JavaScript. While this works for simple cases, it becomes inefficient as the complexity of the application grows. Adding, modifying, or removing elements in a large application required manual DOM manipulations, which could lead to bugs and poor performance.
The inefficiency stems from the fact that the browser doesn’t know which part of the DOM has changed. When a change occurs, it re-renders the entire page or large sections of it, even if only a small part was updated.
This is where the Virtual DOM comes in.
Enter the Virtual DOM
The Virtual DOM (VDOM) is a lightweight in-memory representation of the actual DOM. It mirrors the structure of the real DOM as a tree of JavaScript objects. React uses this VDOM to track changes and efficiently update the real DOM.
Example of a Virtual DOM Node
// Example
let vdom = {
type: 'p',
props: {
class: 'big',
children: [
'Asalamu alaykum!'
]
}
}
In this example, the VDOM node represents a <p>
element with a class attribute and text content. This structure makes it easy for React to compare versions of the VDOM and determine what needs to change in the real DOM.
How Does the Virtual DOM Work?
- Render the VDOM: React creates a Virtual DOM tree based on your React components.
- Diffing: When the application state changes, React creates a new VDOM tree and compares it with the previous VDOM tree (a process called “diffing”).
- Patch the DOM: React calculates the minimal set of changes needed and updates only the parts of the real DOM that have changed.
This process minimizes the number of direct DOM manipulations, improving performance and making state updates predictable.
Updating the VDOM in React
React uses helper functions like createElement to define and update the VDOM. For example:
import { createElement, render } from "https://esm.sh/preact";
const salam = createElement(
"h1",
{ class: "test" },
"Asalamu Alaykum",
);
render(salam, root);
This function creates a VDOM representation of an <h1>
element. When we call render, React (or Preact, in this case) compares the current VDOM with the previous one and applies the necessary updates to the real DOM.
Remeber this from the entry point in a react app? This is where it comes from. We’re basically just adding in 1 large object when initializing the app in most react apps.
This makes more sense now right? React is just a library that helps us manage a VDOM and update the actual DOM efficiently. That’s it! We really did overcomplicate things, especially for beginners…
Conclusion
React abstracts away the complexity of DOM manipulations. When you update the application state in React, you’re essentially creating a new VDOM. React takes care of comparing it with the previous VDOM and applying the changes efficiently. This declarative approach makes your code easier to read and maintain.
Hope this helps in the understanding of the Virtual DOM!